Point Clouds
Turn a depth buffer into a world-space point cloud.
Overview
This feature takes a DepthBuffer and uses ComputeBuffer
s to turn it into a world-space point cloud ready for use in your Unity scene.
The below example of this shows a debug visualization of what a single frame point cloud may look like. Not every point in the cloud is rendered in the demo for performance reasons, just enough to provide a visualization of what it looks like.
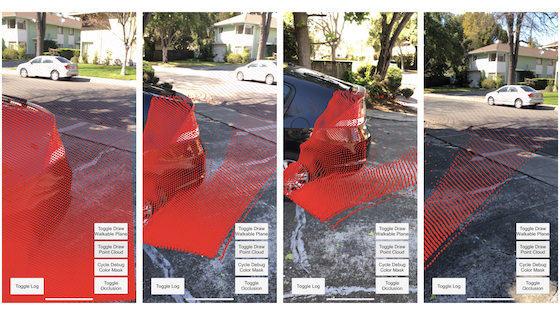
Enabling Point Clouds
In your AR configuration set the IsDepthEnabled
value to true, and enable point cloud generation by setting the IsDepthPointCloudEnabled
property of your ARWorldTrackingConfiguration
to true. After doing this, the point cloud will be available on any ARFrame that has a Depth
value, and can be accessed through the ARFrame
‘s DepthPointCloud property.
using Niantic.ARDK.AR.Configuration; private void RunWithPointCloud(IARSession arSession) { var configuration = ARWorldTrackingConfigurationFactory.Create(); configuration.IsDepthEnabled = true; configuration.DepthTargetFrameRate = 20; configuration.IsDepthPointCloudEnabled = true; arSession.Run(configuration); arSession.FrameUpdated += OnFrameUpdated; } private void OnFrameUpdated(FrameUpdatedArgs args) { var frame = args.Frame; if (frame.Depth != null) { // You can now access the depth point cloud data var depthFeaturePoints = frame.DepthPointCloud; // insert your code for usage of the point cloud } }
Note
To use this feature, you must enable depth as well in your ARSession configuration, since point clouds are built using depth data.